I spent most of October and November building a dog barn for the dogs. Our two newest dogs (Lennier and Monte) don’t have sufficient winter coats to be outside when it’s colder than ‒15°F. A dog barn is a heated space with large, comfortable, locking dog boxes inside. The dogs sleep inside at night and are pretty much in the house with us when we’re home, but when we’re at work or out in town, the dogs can go into the barn to stay warm on cold days.
You can view the photos of the construction on my photolog
Along with the dog boxes we’ve got a monitoring and control system in the barn:
- An Arduino board that monitors the temperature (DS18B20 sensor) and humidity (SHT15) in the barn and controls an electric heater through a Power Tail II.
- A BeagleBone Black board running Linux which reads the data from the Arduino board and inserts it into a database, and can change the set temperature that the Arduino uses to turn the heater on and off (typically we leave this set at 30°F, which means the heater comes on at 28 and goes off at 32°F).
- An old Linksys WRT-54G router (running DD-WRT) which connect to the wireless network in the house and connects to BeagleBone setup via Ethernet.
The system allows us to monitor the conditions inside the barn in real-time, and to change the temperature. It is a little less robust than the bi-metallic thermostat we were using initially, but as long as the Arduino has power, it is able to control the heat even if the BeagleBone or wireless router were to fail, and is far more accurate. It’s also a lot easier to keep track of how long the heater is on if we’re turning it on and off with our monitoring system.
Thursday we got an opportunity to see what happens when all the dogs are in there at ‒15°F. They were put into their boxes around 10 AM, and went outside at 3:30 PM. The windows were closed.
Here’s a series of plots showing what happened (PDF version)
The top plot shows the temperature in the barn. As expected, the temperature varies from 28°F, when the heater comes on, to a bit above 32°F when the heater goes off. There are obvious spikes in the plot when the heater comes on and rapidly warms the building. Interestingly, once the dogs were settled into the barn, the heater didn’t come on because the dogs were keeping the barn warm themselves. The temperature gradually rose while they were in there.
The next plot is the relative humidity. In addition to heating the barn, the dogs were filling it with moisture. It’s clear that we will need to deal with all that moisture in the future. We plan on experimenting with a home-built heat recovery ventilator (HRV) that is based on alternating sheets of Coroplast plastic. The idea is that warm air from inside travels through one set of layers to the outside, cold air from outside passes through the other set of layers and is heated on it’s way in by the exiting warm air. Until that’s done, our options are to leave the two windows cracked to allow the moisture to escape (with some of the warm air, of course) or to use a dehumidifier.
The bar chart shows the number of minutes the power was on for the interval shown. Before the dogs went into the barn the heater was coming on for about 15 minutes, then was off for 60 minutes before coming back on again. As the temperature cools outside, the interval when the heater is off decreases. Again, this plot shows the heater stopped coming on once the dogs were in the barn.
The bottom plot is the outside temperature.
So far the barn is a great addition to the property, and the dogs really seem to like it, charging into the barn and into their boxes when it’s cold outside. I’m looking forward to experimenting with the HRV and seeing what happens under similar conditions but with the windows slighly open, or when the outside temperatures are much colder.
After writing my last blog post I decided I really should update the code so I can control the temperature thresholds without having to rebuild and upload the code to the Arduino. That isn’t a big issue when I have easy access to the board and a suitable computer nearby, but doing this over the network is dangerous because I could brick the board and wouldn’t be able to disconnect the power or press the reset button. Also, the default position of the transistor is off, which means that bad code loaded onto the Arduino shuts off the fan.
But I will have a serial port connection through the USB port on the server (which is how I will monitor the status of the setup), and I can send commands at the same time I’m receiving data. Updating the code to handle simple one-character messages is pretty easy.
First, I needed to add a pair of variables to store the thresholds, and initialize them to their defaults. I also replaced the hard-coded values in the temperature difference comparison section of the program with these variables.
int temp_diff_full = 6;
int temp_diff_half = 2;
Then, inside the main loop, I added this:
// Check for inputs
while (Serial.available()) {
int ser = Serial.read();
switch (ser) {
case 70: // F
temp_diff_full = temp_diff_full + 1;
Serial.print("full speed = ");
Serial.println(temp_diff_full);
break;
case 102: // f
temp_diff_full = temp_diff_full - 1;
Serial.print("full speed = ");
Serial.println(temp_diff_full);
break;
case 72: // H
temp_diff_half = temp_diff_half + 1;
Serial.print("half speed = ");
Serial.println(temp_diff_half);
break;
case 104: // h
temp_diff_half = temp_diff_half - 1;
Serial.print("half speed = ");
Serial.println(temp_diff_half);
break;
case 100: // d
temp_diff_full = 6;
temp_diff_half = 2;
Serial.print("full speed = ");
Serial.println(temp_diff_full);
Serial.print("half speed = ");
Serial.println(temp_diff_half);
break;
}
}
This checks to see if there’s any data in the serial buffer, and if there is, it reads it one character at a time. Raising and lowering the full speed threshold by one degree can be done by sending F to raise and f to lower; the half speed thresholds are changed with H and h, and sending d will return the values back to 6 and 2.
One of our servers at work has a power supply that is cooled with two small, loud fans—loud enough that the noise is annoying for the occupants of the office suite shared by the server. What I’d like to do is muffle the sound by enclosing the rear of the server (or potentially just the power supply exhaust) with an actively vented box. Since the fan on the box will be larger, it should be able to move the same amount of air with less noise.
But messing around with the way servers cool themselves is tricky, and since the server isn’t easily accessible, I’d like to be able to monitor what’s going on until I confirm my setup is able to keep the server cool.
I’ve built quite a few temperature monitoring circuits, but in this project I’ll need to be able to control the speed of the vent fan based on the temperature differential inside and outside the box. This is complicated because the Arduino runs on 5 volts and the fan I’m starting with requires 12 volts. I’ll use a Darlington transistor, which can be triggered using the low power available from an Arduino pin, but which can carry the voltage and current of the fan. For higher voltages and currents, or for controlling devices that run on alternating current, I’d need to use some form of relay.
To measure the temperature differential between the inside and outside of the enclosure, I’m using two DS18B20 temperature sensors, each wired to the end of a length of Cat5e cable (and wrapped in silicone “Rescue Tape” as an experiment). The transistor is a TIP120, and a 1N4001 diode across the positive and negative leads of the fan protect it from reverse voltages when the fan is turned off but is still spinning. A 2.2KΩ resistor protects the trigger pin on the Arduino.
Here’s the diagram:
The Arduino is currently programmed to turn the fan on full speed when the temperature from the sensor inside the box is more than six degrees higher than the temperature outside the box. The fan runs at half speed until there’s less than a two degree differential, at which point the fan shuts off. These targets are hard-coded in the software, but it wouldn’t be too difficult to change the code to read from the serial buffer so that the thresholds could be changed while it’s running.
Here’s the setup code:
#include <OneWire.h>
#include <DallasTemperature.h>
#define ONE_WIRE_BUS 8
#define TEMPERATURE_PRECISION 12
#define fanPin 9
OneWire oneWire(ONE_WIRE_BUS);
DallasTemperature sensors(&oneWire);
DeviceAddress thermOne = { 0x28,0x7A,0xBA,0x0A,0x02,0x00,0x00,0xD1 };
DeviceAddress thermTwo = { 0x28,0x8B,0xFD,0x0A,0x02,0x00,0x00,0x96 };
void setup() {
Serial.begin(9600);
pinMode(fanPin, OUTPUT);
digitalWrite(fanPin, LOW);
sensors.begin();
sensors.setResolution(thermOne, TEMPERATURE_PRECISION);
sensors.setResolution(thermTwo, TEMPERATURE_PRECISION);
}
void printTemperature(DeviceAddress deviceAddress) {
float tempC = sensors.getTempC(deviceAddress);
Serial.print(DallasTemperature::toFahrenheit(tempC));
}
And the loop that does the real work:
void loop() {
sensors.requestTemperatures();
printTemperature(thermOne);
Serial.print(",");
printTemperature(thermTwo);
Serial.print(",");
float thermOneF = DallasTemperature::toFahrenheit(sensors.getTempC(thermOne));
float thermTwoF = DallasTemperature::toFahrenheit(sensors.getTempC(thermTwo));
float diff = thermOneF - thermTwoF;
Serial.print(diff);
Serial.print(",");
if ((diff) > 6.0) {
Serial.println("high");
digitalWrite(fanPin, HIGH);
} else if (diff > 2.0) {
Serial.println("med");
analogWrite(fanPin, 127);
} else {
Serial.println("off");
digitalWrite(fanPin, LOW);
}
delay(10000);
}
When I’m turning the fan off or on, I’m using digitalWrite, but when running the fan at half-speed, because I’m using a pulse-width-modulation (PWM) digital pin, I can set the pin to a value between 0 (off all the time) and 255 (on all the time) and cause the fan to run at whatever speed I want. I’m also dumping the temperatures and fan speed setting to the serial port so I can read those values later and evaluate how well the setup is working.
Last week I got permission to install a simple weather station where I work at ABR. The Fairbanks office is located on Goldstream Road, just past the intersection of Murphy Dome Road, across the street from the Peat Ponds. It’s on the uphill side of a south facing slope, and is about half a mile from Goldstream Creek. More importantly for me, I can find out what the temperature is at work when I’m dressing for riding my bike or skiing.
We ran three Cat5e cables from the computer room, outside and under a footpath, then down the hill from the Main building. The cables end up in a mixed spruce-birch forest, and the sensors will be about six feet off the ground in one of those cylindrical “white plate” style enclosures.
I’m using a pair of DS18B20 temperature sensors, which have a 0.5°C accuracy across their range, and will work down to -67°F. The data is retrieved using the One-Wire protocol, which is well supported by Arduino. I built a prototype of the sensor before soldering it down on a board because longer distances can require a smaller pull-down resistor than the 4.7KΩ typically used between the voltage and data lines. I also wanted to experiment with whether I could run two using parasitic power, or would need two data lines. As it turned out, 4.7KΩ worked, as did parastic power mode.
The schematic is below, including the wires I used in the Cat5e cable. I followed the PoE standard, even though I could really have used whatever wires I wanted.
I also used a contributed library called DallasTemperature which abstracts most of the work of using OneWire and converting the values into meaningful temperatures. Here's the setup code:
#include <OneWire.h>
#include <DallasTemperature.h>
#define ONE_WIRE_BUS 8
#define TEMPERATURE_PRECISION 9
OneWire oneWire(ONE_WIRE_BUS);
DallasTemperature sensors(&oneWire);
// Use a testing program to find your addresses:
DeviceAddress thermOne = {
0x28, 0xBC, 0x29, 0xE3, 0x02, 0x00, 0x00, 0xE1 };
DeviceAddress thermTwo = {
0x28, 0xCF, 0x97, 0xE3, 0x02, 0x00, 0x00, 0xCD };
void setup(void) {
Serial.begin(9600);
sensors.begin();
sensors.setResolution(thermOne, TEMPERATURE_PRECISION);
sensors.setResolution(thermTwo, TEMPERATURE_PRECISION);
}
And the part that does the work:
void printTemperature(DeviceAddress deviceAddress) {
float tempC = sensors.getTempC(deviceAddress);
Serial.print(DallasTemperature::toFahrenheit(tempC));
}
void loop(void) {
sensors.requestTemperatures();
printTemperature(thermOne);
Serial.print(",");
printTemperature(thermTwo);
Serial.println();
delay(1000);
}
Here’s the schematic. The top form shows two sensors in parasitic power mode (where you only need a single data line, power and ground), and the bottom form shows how you’d wire a single sensor. If I had trouble with getting reliable data from the sensors in parasitic mode, or with a 4.7KΩ resistor, I would have dropped the resistor to 3.3KΩ or 2.2KΩ. The next thing I would have tried would be running power and ground to both sensors separately (coming from wires 4 and 7 in the cable), and used wire 1 and wire 2 for the data lines to each sensor. As it turned out, I’m only using three of the eight wires in the cable.
Update 2012-10-15: I moved the resistor from the sensor board (you can see it in the photograph at the top of the page) to the Arduino end of the cable inside ABR, and the data is still flowing. I was a little concerned that the resistor might be adding a small amount of heat to the enclosure, and putting it at the Arduino side prevents that possibility.
Update 2012-10-19: Today I added a third DS18B20 in parasitic mode and I’m getting data from all three sensors. I would guess the total cable run is around 100 feet from the Arduino to the sensors. So: 100 feet of Cat 5e, three sensors in parasitic mode, with a 4.7KΩ pull-down resistor between power and data on the Arduino side of the cable.
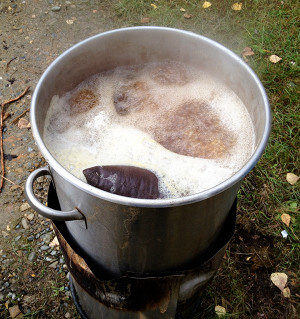
Boil
Over the past month and a half I’ve brewed four beers, starting with Piper’s Irish-American Ale , and culminating with Mr. Silly IPA which is in the middle of the mash right now. We’re less than a week from when we normally get the first snowfall that lasts until spring, so this will likely be the end of my 2012 brewing effort.
My normal process is to make a yeast starter from a Wyeast smack-pack or White Labs tube, pitch that into the first batch, and a week later siphon the chilled wort of the second batch onto the yeast cake from the first.
I didn’t have time to make a starter for Piper’s, so I just smacked the pack (Wyeast 1084, Irish Ale) on brew day and assumed there’d be enough healthy yeast to make quick work of the 1.049 wort. After two days of no visible activity, I began to worry that the yeast in the pack had been killed at some point before I bought it. Finally on day three, something finally started to happen and the beer has since fermented successfully.
The second batch was a 1.077 old ale I poured into my Old Alexi solera ale keg (recipe is here). It kicked off almost immediately and was probably 90% fermented within 24 hours due to the thick, healthy yeast from fermenting Piper’s.
During both fermentations I kept track of the temperatures in the fermentation chamber (a fridge with heater on a temperature controller) and in the wort using my Arduino data logger. A graph of the two fermentations is below:
You can see the activity of the temperature controller on the temperature in the chamber (the orange line), clicking the heater on and off within 4 degrees of the set temperature. For Piper’s (the top plot) I started with the chamber at 64°F, but almost immediately bumped it up to 66°F when the wort temperature crashed. After 24 hours, something finally started happening in the wort, with a peak temperature (and fermentation) on day three. When I transferred the wort from primary to secondary on day seven, there was still active fermentation.
Compare this with the second beer. The wort started at 65°F, and immediately took off, peaking a little over 24 hours later. By day three, fermentation was over. I dropped the fermentation chamber temperature from 66°F to 64°F after the first day.
What did I learn? First: always make a yeast starter. It’s no fun waiting several days for fermentation to finally take off, and during that lull the wort is vulnerable to other infections that could damage the flavor. Second: don’t panic, especially with a yeast that has a reputation of starting slowly like Wyeast 1084. It usually works out in the end. More often than not, the Papazian “relax, enjoy a homebrew” mantra really is the way to approach brewing.
I used a starter for last week’s batch (Taiga Dog AK Mild, White Labs WLP007, Dry English Ale) and it was visibly fermenting within a day and a half. Mr. Silly will undoubtedly have a similar fermentation temperature curve like Old Alexi above after I transfer the wort onto the Taiga Dog yeast cake.